The method getDeviceId() of TelephonyManager returns the unique device ID, for example, the IMEI for GSM and the MEID or ESN for CDMA phones. Return null if device ID is not available.
The Result is:
Java code :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
| package com.AndroidTelephonyManager; import android.app.Activity; import android.content.Context; import android.os.Bundle; import android.telephony.TelephonyManager; import android.widget.TextView; public class AndroidTelephonyManager extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super .onCreate(savedInstanceState); setContentView(R.layout.main); TextView textDeviceID = (TextView)findViewById(R.id.deviceid); //retrieve a reference to an instance of TelephonyManager TelephonyManager telephonyManager = (TelephonyManager)getSystemService(Context.TELEPHONY_SERVICE); textDeviceID.setText(getDeviceID(telephonyManager)); } String getDeviceID(TelephonyManager phonyManager){ String id = phonyManager.getDeviceId(); if (id == null ){ id = "not available" ; } int phoneType = phonyManager.getPhoneType(); switch (phoneType){ case TelephonyManager.PHONE_TYPE_NONE: return "NONE: " + id; case TelephonyManager.PHONE_TYPE_GSM: return "GSM: IMEI=" + id; case TelephonyManager.PHONE_TYPE_CDMA: return "CDMA: MEID/ESN=" + id; /* * for API Level 11 or above * case TelephonyManager.PHONE_TYPE_SIP: * return "SIP"; */ default : return "UNKNOWN: ID=" + id; } } } |
XML Code:
1
2
3
4
| <linearlayout android:layout_height= "fill_parent" android:layout_width= "fill_parent" android:orientation= "vertical" xmlns:android= "http://schemas.android.com/apk/res/android" > <textview android:layout_height= "wrap_content" android:layout_width= "fill_parent" android:text= "@string/hello" > <textview android:id= "@+id/deviceid" android:layout_height= "wrap_content" android:layout_width= "fill_parent" > </textview></textview></linearlayout> |
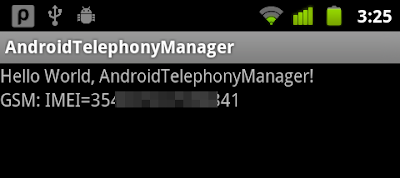
You'll need the
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
permission to do this in menifest..
No comments:
Post a Comment